In the fast-paced world of web development, optimizing performance is crucial to delivering a seamless user experience. One key aspect of this optimization is reducing the bundle size of your web application. In this article, we will explore why smaller bundle sizes are important, how to analyze your bundle using webpack, strategies to improve bundle size, and proactive measures to prevent bloating in the future.
Why smaller bundle size is important?
Smaller bundle sizes contribute directly to faster page load times, a critical factor in retaining user engagement. Users today expect instant responses, and a large bundle size can lead to slower load times, resulting in potential bounce rates and a negative impact on user satisfaction.
Additionally, smaller bundles benefit users with limited bandwidth, especially on mobile devices. Faster load times not only improve user experience but also positively influence search engine rankings, making it essential for any web application.
How to analyze your bundle with Webpack?
Understanding your current bundle composition is the first step in optimization.
Webpack provides powerful tools to analyze and visualize your bundle.
Tools like webpack-bundle-analyzer offer an insightful breakdown of dependencies and their sizes. By identifying large dependencies or unnecessary duplicates, you can pinpoint areas for improvement.
To use webpack-bundle-analyzer, simply install it using npm:
npm install --save-dev webpack-bundle-analyzer
Then, add it to your webpack configuration:
const BundleAnalyzerPlugin =
require("webpack-bundle-analyzer").BundleAnalyzerPlugin;
module.exports = {
// ... other configurations
plugins: [new BundleAnalyzerPlugin()],
};
Last thing is to build your application either via a script in your package.json or by running the following command in the root directory which will open interactive visualization of your bundle structure at http://localhost:8888:
npx webpack
How did we reduce our bundle size by 40%?
Enough with the boring stuff. Let's get straight to the point HOW TO?
First to get a perspective here is how the visualization of your bundle will look like
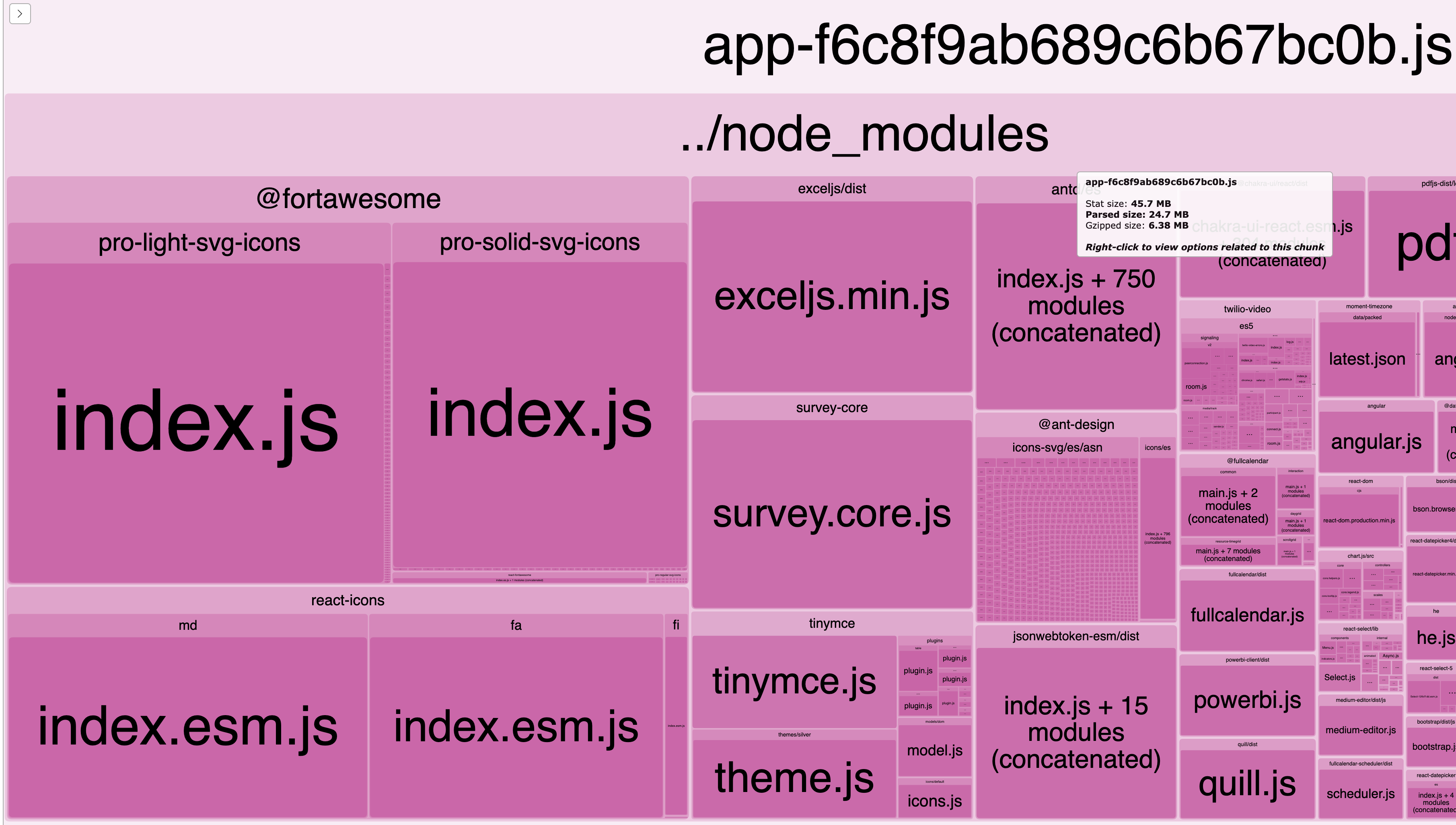
We had an ENORMOUS 6.38MB(yes - MB!) bundle size to work with. So we identified three main things we can easily fix:
- Tree-shaking @fortawesome doesn't work so we had to fix the deep import
From:
import { faCoffee } from "@fortawesome/free-solid-svg-icons";
To:
import { faCoffee } from "@fortawesome/free-solid-svg-icons/faCoffee";
-
Tree shaking react-icons doesn't work as well so we removed it and switched to using @fortawesome. That shaved 300KB alone!
-
We replaced jsonwebtoken with jwt-decode as it was used in the browser and we don't need to bundle all of the Node stuff from jsonwebtoken
With just those 3 changes we managed to get the bundle size to 3.8MB
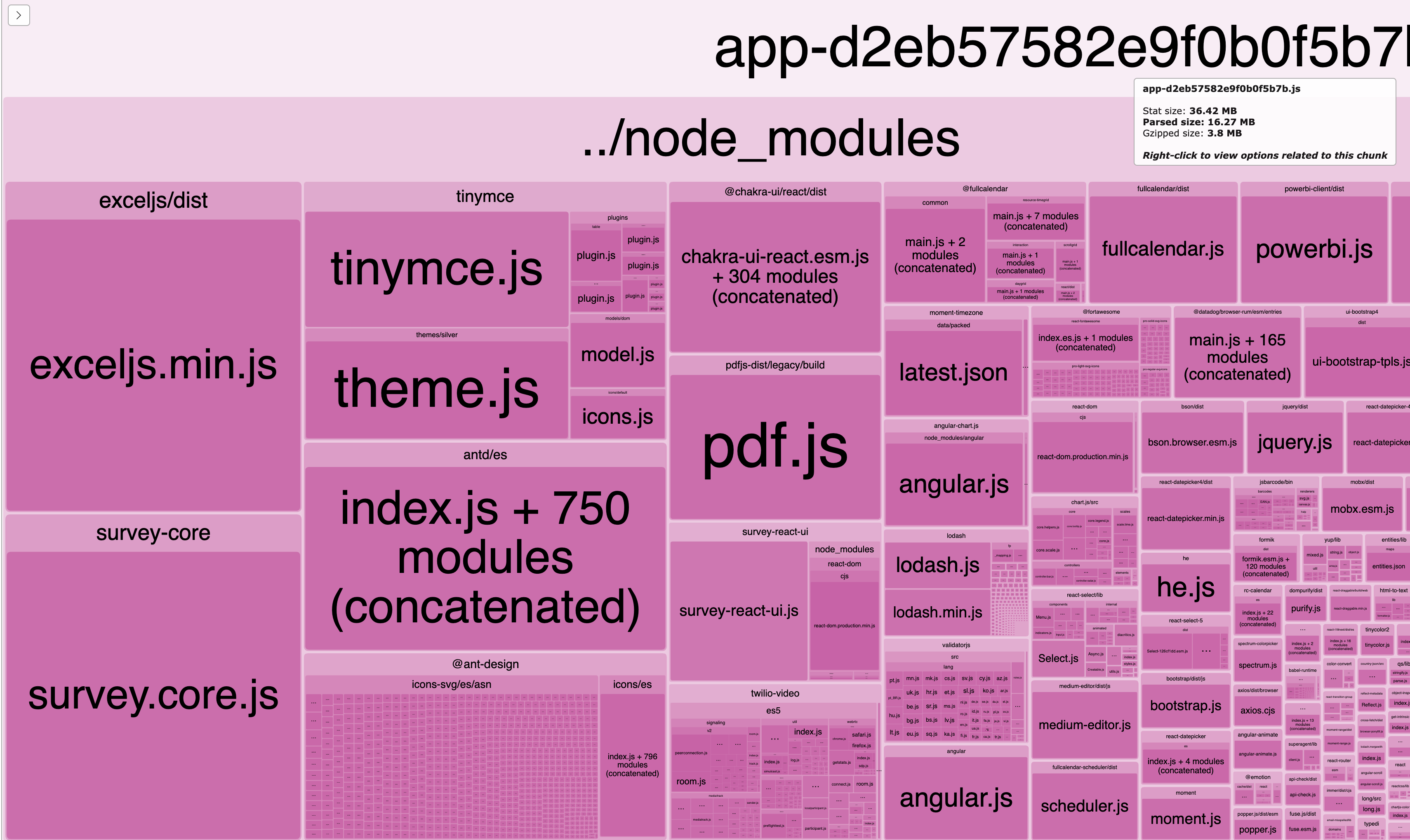
As you can see there is still room for improvement 😉
What else can you do to improve the bundle size?
-
Code Splitting: Utilize code splitting to break your application into smaller chunks, loading only the necessary code for each specific page or feature. This can be achieved using dynamic imports or Webpack's built-in SplitChunksPlugin. Code splitting reduces the initial load size and speeds up subsequent loads by loading only what is needed.
-
Tree Shaking: Take advantage of tree shaking, a feature that eliminates unused code during the bundling process. This is particularly effective with modern JavaScript frameworks like React or Vue, where unused components or functions can be automatically excluded from the final bundle.
-
Optimize Images and Assets: Compress and optimize images and other assets to reduce their size. Tools like image-minimizer-webpack-plugin can be integrated into your webpack configuration to automatically optimize images during the build process.
How to prevent bundle size bloatin in the future?
-
Continuous Monitoring: Regularly monitor your bundle size using tools like webpack-bundle-analyzer to identify any unexpected increases. Set up continuous integration (CI) pipelines to catch regressions early in the development process.
-
Bundle Size Budgets: Establish bundle size budgets for your application and enforce them using tools like size-limit. This ensures that developers are aware of the impact of their changes on the overall bundle size.
-
Dependency Management: Keep dependencies in check and regularly update them to their latest versions. Some updates might include performance improvements or reduced bundle sizes. Utilize tools like
npm audit
to identify and address security vulnerabilities in your dependencies.